Operations
Align
After creating multiple objects, align
allows you to align an array of objects:
const shapes = [
cube({center: [0, 0, 10]}),
cube({center: [0, 10, 20]}),
cube({center: [10, 0, 30]})
];
const alignedShapes = align(
{
modes: ['center', 'max', 'none'], // align along axis: center, min, max, none
realtiveTo: [0,0,0]
},
shapes
);
Boolean Operations
const {union, subtract, intersect, scission} = jscad.booleans;
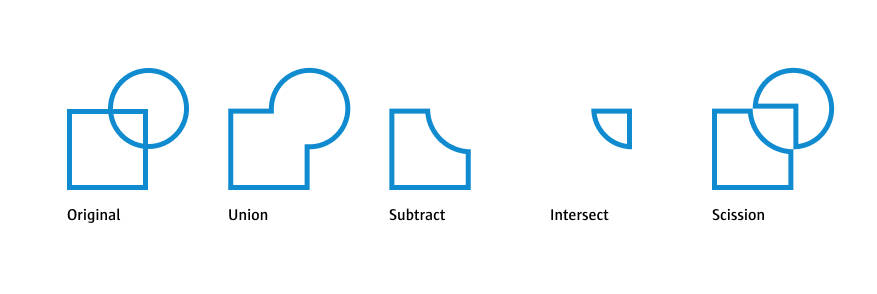
Union
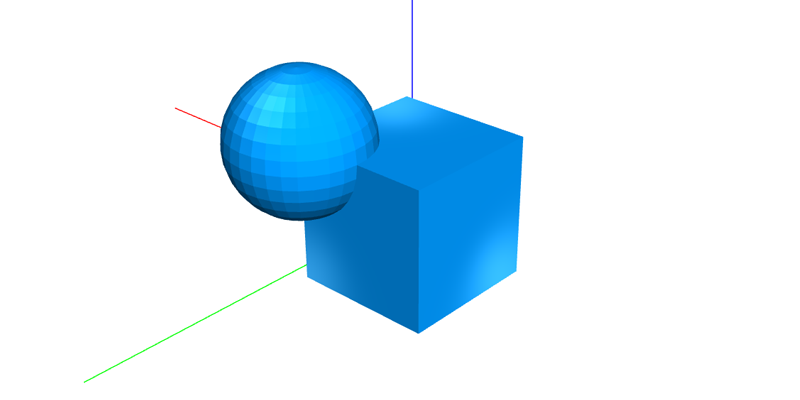
Combines an array of geometries into one new geometry:
const shapes = [
cube({size: 4}),
sphere({radius: 2, center: [2, 2, 2]})
];
const unionShape = union(shapes);
return unionShape;
Subtract
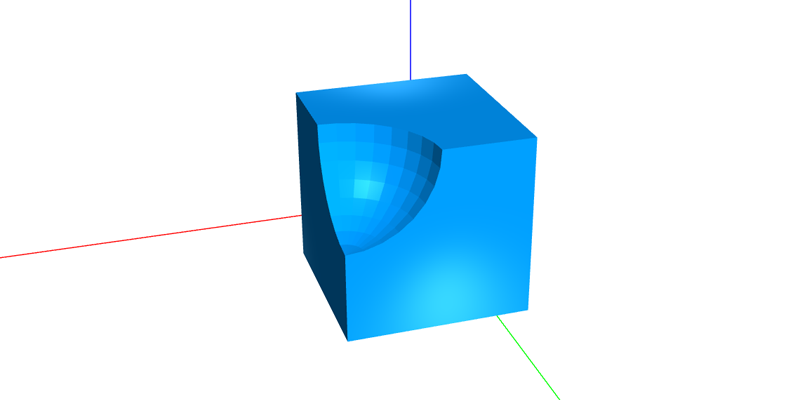
Cut out shapes from the first shape in the list:
const shapes = [
cube({size: 4}),
sphere({radius: 2, center: [2, 2, 2]})
];
const subtractShape = subtract(shapes);
return subtractShape;
Intersect
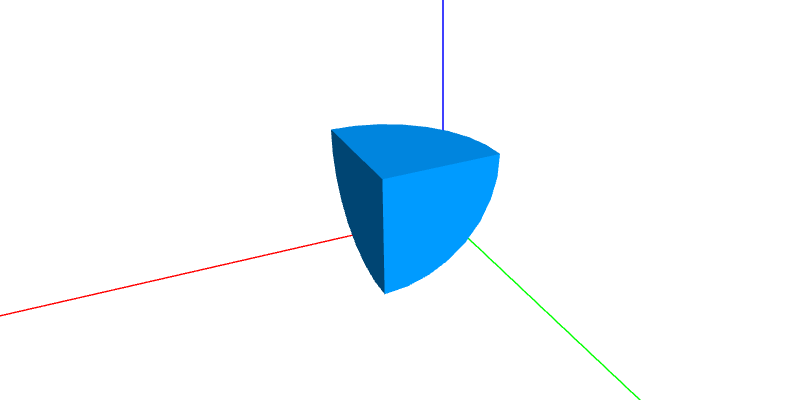
The overlapping areas of the object in the array:
const shapes = [
cube({size: 4}),
sphere({radius: 2, center: [2, 2, 2]})
];
const intersectShape = intersect(shapes);
return intersectShape;
Scission
Scission can cut elements apart that are not connected anymore. This requires that there is at least a little gap inbetween two elements. If one for example would use subtract
on two shapes in both directions and afterwards union
. The cut would be so perfect, that the edges would still touch. Here is an example where the shapes are translated before union
is applied, thereby, creating a big gap:
const cubeShape = cube({size: 4});
const sphereShape = sphere({radius: 2, center: [2, 2, 2]});
const cut1 = subtract([cubeShape, sphereShape]);
const cut2 = subtract([sphereShape, cubeShape]);
const unionShape = union([
translate([0,0,0], cut1),
translate([0,0,5], cut2)
]);
const scissionShapes = scission(unionShape);
The above scission
returns an array with two elements, which are the object that went into the union
command.
Task: Union & Subtract
First create an object by combining multiple 3D bodies through **union**. Then use *subtract* to remove bits from the union-object.
Inspiration: Raspberry Pi Case
Based on the mechanical drawings of the Raspberry Pi, particularly the screw holes, this case is constructed. Using variables the size can be easily modified.
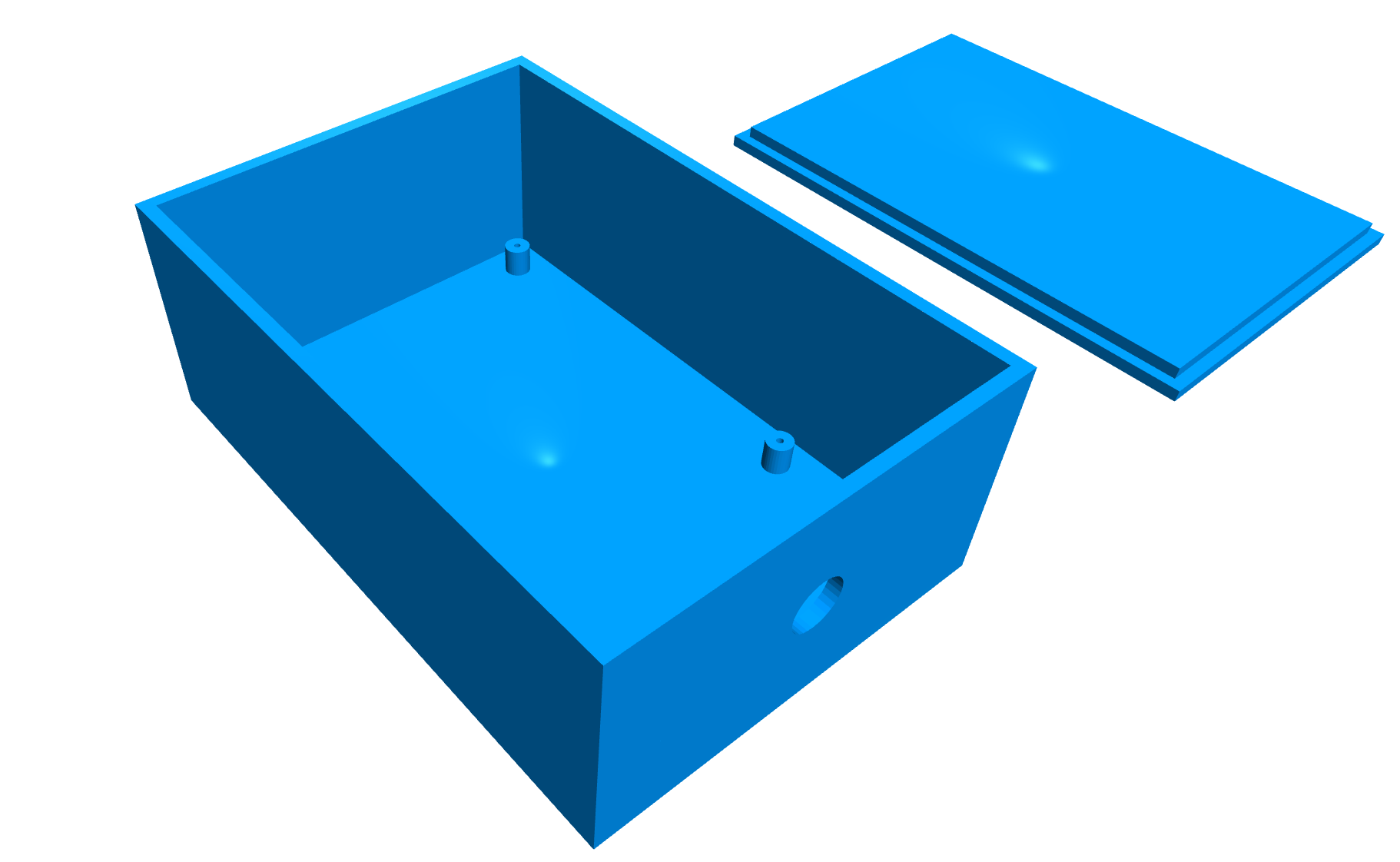
View on GitHub/lectures/3d/case